반응형
객체지향프로그래밍 8장 제네릭과 컬렉션
실습문제 2번 10개의 나라 이름과 인구 수를 입력받고 나라 이름을 검색하여 인구 수 출력하기 (해시)
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
|
import java.util.Hashtable;
import java.util.Scanner;
public class list {
public static void main(String[] args) {
Hashtable<String, Integer> nations = new Hashtable<String, Integer>();
Scanner s = new Scanner(System.in);
for(int a = 1; a<=10; a++){
System.out.print(a+"번째 나라이름 :");
String s1 = s.next();
System.out.print("인구 수:");
Integer i1 = s.nextInt();
nations.put(s1, i1);
}
System.out.print("검색할 나라이름 입력:");
String s1 = s.next();
System.out.println(nations.get(s1));
}
}
|
cs |
실습문제 4번 사각형 클래스와 선분 클래스에서 백터 활용하기
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
|
import java.util.Vector;
abstract class GraphicObject {
protected int x,y,w,h;
public GraphicObject(int x, int y, int w, int h){
this.x = x;
this.y = y;
this.w = w;
this.h = h;
}
public abstract void view();
}
class Rect extends GraphicObject{
public Rect(int x, int y, int w, int h){
super(x, y, w, h);
}
public void view(){
System.out.println(x+","+y+"->"+w+","+h+"의 사각형");
}
}
class Line extends GraphicObject{
public Line(int x, int y, int w, int h){
super(x, y, w, h);
}
public void view(){
System.out.println(x+","+y+"->"+w+","+h+"의 선");
}
}
public class GraphicEditor{
private Vector<GraphicObject> v;
public GraphicEditor(){
v = new Vector<GraphicObject>();
}
void add(GraphicObject g){
v.add(g);
}
void draw(){
for(int i = 0; i<v.size(); i++){
v.get(i).view();
}
}
public static void main(String[] args) {
GraphicEditor g = new GraphicEditor();
g.add(new Rect(2,2,3,4));
g.add(new Line(3,3,8,8));
g.add(new Line(2,5,6,6));
g.draw();
}
}
|
cs |
실습문제 5번 탬플릿 형변환 사용해보기
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
|
public class MyClass<T> {
private T s;
public MyClass(T s){
this.s = s;
}
void setS(T s){
this.s = s;
}
T getS(){
return s;
}
public static void main(String[] args) {
MyClass my = new MyClass (new Integer(10));
System.out.println(my.getS());
my.setS(new Double(3.14));
System.out.println(my.getS());
}
}
|
cs |

실습문제 6번 ArrayList 활용해보기
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
|
import java.util.ArrayList;
import java.util.Scanner;
public class MyClass{
private String name;
private String dept;
private int id;
private double avg;
public MyClass(String name, String dept, int id, double avg){
this.name = name;
this.dept = dept;
this.id = id;
this.avg = avg;
}
public void view(){
System.out.println("이름: "+name+"\t학과: "+dept+
"\n학번: "+id+"\t\t학점 평균: "+avg);
}
public static void main(String[] args) {
Scanner s = new Scanner(System.in);
ArrayList<MyClass> a= new ArrayList<MyClass>();
for(int i = 0; i<5; i++){
String Name = s.next();
String Dept = s.next();
int Id = s.nextInt();
double Avg = s.nextDouble();
MyClass my = new MyClass(Name,Dept,Id,Avg);
a.add(my);
}
for(int i = 0; i<a.size(); i++){
a.get(i).view();
}
}
}
|
cs |
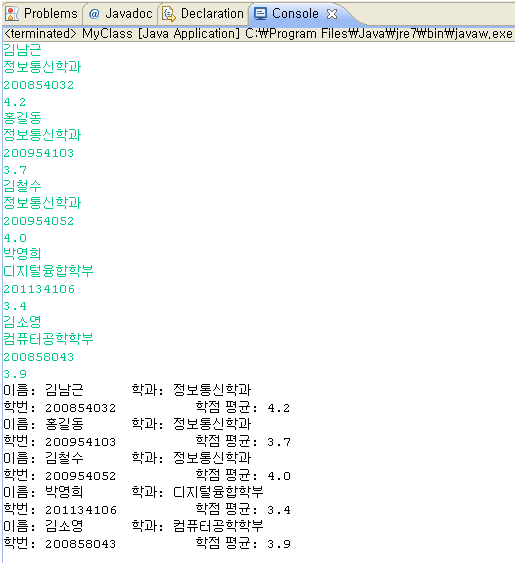
반응형
'학습공간 > JAVA, 객체지향프로그래밍' 카테고리의 다른 글
[7주차] 자바의 이벤트 처리 (0) | 2019.11.28 |
---|---|
[6주차] GUI 실습 (0) | 2019.11.28 |
[4주차] 상속 (0) | 2019.11.28 |
[3주차] 클래스와 객체 (0) | 2019.11.28 |
[2주차] 반복문과 배열 그리고 예외처리 (0) | 2019.11.28 |