반응형
이제부터 본격적으로 자바 객체 지향 프로그래밍 실습의 시작이다.
아래와 같이 메인함수에서 어느 정도 익숙해진 상태라면 클래스 함수를 직접 구현해보고 사용해보자.
public class ClassName {
public ClassFunction1() {
}
public static void main(String[] args) {
/* write code here */
ClassName []obj = new ClassName [5];
obj[0] = new ClassName();
obj[0].ClassFunction1();
}
}
예제 1 수강과목 5개 입력받고 출력하기
실습문제 1번 앨범 정보 프로그램
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
|
public class Song { // Song.java
public static void main(String[] args) {
Song2 a = new Song2("Dancing Queen", "ABBA");
String [] jak= new String [5];
a.show();
jak[0] = "작곡가 1";
jak[1] = "작곡가 2";
a.setTitle("새 노래제목");
a.setArtist("아티스트");
a.setAlbum("새 앨범");
a.setComposer(jak);
a.setYear(2001);
a.setTrack(9);
a.show();
}
}
public class Song2 { // Song2.java
private String title;
private String artist;
private String album;
private String [] composer;
private int year;
private int track;
public String getTitle() {
return title;
}
public void setTitle(String title) {
this.title = title;
}
public String getArtist() {
return artist;
}
public void setArtist(String artist) {
this.artist = artist;
}
public String getAlbum() {
return album;
}
public void setAlbum(String album) {
this.album = album;
}
public String[] getComposer() {
return composer;
}
public void setComposer(String[] composer) {
this.composer = composer;
}
public int getYear() {
return year;
}
public void setYear(int year) {
this.year = year;
}
public int getTrack() {
return track;
}
public void setTrack(int track) {
this.track = track;
}
Song2 (String title, String artist ){
this.title = title;
this.artist = artist;
album = "앨범";
composer = new String [5];
year = 1996;
track = 7;
composer[0] = "작곡가1";
}
public void show(){
System.out.println(title);
System.out.println(artist);
System.out.println(album);
for(int i =0; composer[i] != null; i++)
System.out.print(composer[i]+" ");
System.out.println();
System.out.println(year);
System.out.println(track);
System.out.println();
}
}
|
cs |
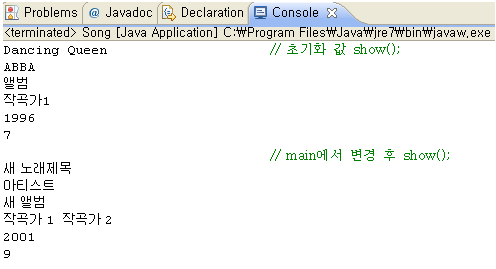
실습문제 5번 덧셈 뺄셈 곱셈 나눗셈 클래스를 쪼개서 별도의 클래스를 생성한 뒤 모두 불러서 사용해보자.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
|
public class Add { // Add.java
private int a, b;
public void setValue(int a, int b){
this.a = a;
this.b = b;
}
public int calculate(){
int result;
result = a+b;
return result;
}
}
public class Sub { // Sub.java
private int a, b;
public void setValue(int a, int b){
this.a = a;
this.b = b;
}
public int calculate(){
int result;
result = a-b;
return result;
}
}
public class Mul { // Mul.java
private int a, b;
public void setValue(int a, int b){
this.a = a;
this.b = b;
}
public int calculate(){
int result;
result = a*b;
return result;
}
}
public class Div { // Div.java
private int a, b;
public void setValue(int a, int b){
this.a = a;
this.b = b;
}
public int calculate(){
int result;
result = a/b;
return result;
}
}
import java.util.Scanner;
public class Calculation { // Calculation.java
public static void main(String[] args) {
int a,b;
String c;
char d;
System.out.print("두 정수와 연산자를 입력하시오>>");
Scanner s = new Scanner(System.in);
a = s.nextInt(); b = s.nextInt();
c = s.nextLine(); d = c.charAt(1);
switch(d)
{
case '+' :
Add add = new Add();
add.setValue(a, b);
System.out.println(add.calculate());
break;
case '-' :
Sub sub = new Sub();
sub.setValue(a, b);
System.out.println(sub.calculate());
break;
case '*' :
Mul mul = new Mul();
mul.setValue(a, b);
System.out.println(mul.calculate());
break;
case '/' :
Div div = new Div();
div.setValue(a, b);
System.out.println(div.calculate());
break;
default :
}
}
}
|
cs |
실습문제 6번 좌석 예약 프로그램(S, A, B 등급 좌석의 갯수는 정해져 있으며, 예약/조회/취소/끝내기 기능을 구현)
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
117
118
119
120
121
122
123
124
125
126
|
public class Seat { // Seat.java
private String name;
Seat() {
name = null;
}
public String getName() {
return name;
}
public void cancel() {
name = null;
}
public void reserve(String name) {
this.name = name;
}
public boolean isOccupied() {
if (name == null) // 좌석이 예약되어 있으면 true 반환
return false;
else
return true;
}
public boolean match(String name) {
return(name.equals(this.name));
}
}
import java.util.Scanner;
public class SeatType { // SeatType.java
private char type;
private Seat[] aSeat;
SeatType(char type, int num) {
this.type = type;
aSeat = new Seat[num];
for (int i=0; i<aSeat.length; i++)
aSeat[i] = new Seat();
}
public boolean reserve() {
/* write code here */
Scanner s = new Scanner(System.in);
System.out.print("이름>>");
String name = s.nextLine();
System.out.print("번호>>");
int number = s.nextInt();
if(!aSeat[number-1].isOccupied()){
aSeat[number-1].reserve(name);
return true;
}
else{
System.out.println("자리가 차 있습니다.");
return false;
}
}
public boolean cancel() {
/* write code here */
Scanner s = new Scanner(System.in);
System.out.print("이름>>");
String name = s.nextLine();
for(int i=0; i<aSeat.length; i++){
if(aSeat[i].match(name)){
aSeat[i].cancel();
return true;
}
}
return false;
}
public void show() {
/* write code here */
System.out.print(type+">> ");
for(int i = 0; i< aSeat.length; i++){
if(!aSeat[i].isOccupied())
System.out.print("--- ");
else
System.out.print(aSeat[i].getName()+" ");
}
System.out.println();
}
}
import java.util.Scanner;
public class Reserve { // Reserve.java
public static void main (String[] args) {
Scanner sin = new Scanner(System.in);
SeatType[] aSeatType = new SeatType[3];
aSeatType[0] = new SeatType('S', 10); // S 타입 좌석 생성
aSeatType[1] = new SeatType('A', 15); // A 타입 좌석 생성
aSeatType[2] = new SeatType('B', 20); // B 타입 좌석 생성
int choice = 0;
while (choice != 4) {
int type;
System.out.print("예약(1), 조회(2), 취소(3), 끝내기(4)>>");
choice = sin.nextInt();
switch (choice) {
case 1: // 예약
System.out.print("좌석구분 S(1), A(2), B(3)>>");
type = sin.nextInt();
if (type < 1 || type > 3) {
System.out.println("잘못된 좌석 타입입니다.");
break;
}
aSeatType[type-1].reserve();
break;
case 2: // 조회
for (int i=0;i<aSeatType.length; i++)
aSeatType[i].show();
System.out.println("<<<조회를 완료하였습니다.>>>");
break;
case 3: // 취소
System.out.print("좌석구분 S(1), A(2), B(3)>>");
type = sin.nextInt();
if (type < 1 || type > 3) {
System.out.println("잘못된 좌석 타입입니다.");
break;
}
aSeatType[type-1].cancel();
break;
case 4: // 끝내기
break;
default:
System.out.println("잘못 입력하셨습니다.");
}
}
}
}
|
cs |
반응형
'학습공간 > JAVA, 객체지향프로그래밍' 카테고리의 다른 글
[6주차] GUI 실습 (0) | 2019.11.28 |
---|---|
[5주차] 제네릭과 컬렉션 (0) | 2019.11.28 |
[4주차] 상속 (0) | 2019.11.28 |
[2주차] 반복문과 배열 그리고 예외처리 (0) | 2019.11.28 |
[1주차] 자바 기본 프로그래밍 (0) | 2019.11.28 |